-
Доставляем заказы по всей РоссииОтправляем во все регионы РФ
Оплата при получении заказа
Есть самовывоз в Москве и в Санкт-ПетербургеДоставка -
Только оригинальные электрошокерыСамый мощный электрошокер Гепард (Ягуар-9)
Электрошокер Удар 2-У Усиленный
Дубинка электрошокер Скипетр X-8
Фонарь электрошокер ОСА 1101
КаталогЭлектрошокеры фонари -
Надоели бродячие собаки?Электрошокер- это лучшее средство от нападения собак. Отпугивает собак на расстоянии до 20 метровКаталог
-
В продаже газовые баллочники- Аэрозольные
- Струйные
- Аэрозольно-струйные
- От животных
- и другиеВыбрать газовый баллончик

Бесплатный звонок
8 800 555 85 62
8 800 555 85 62
- Хит
- Советуем
- Новинка
- Акция
-
-
Мало2 690 руб. /шт4 690 руб.-43%Экономия 2 000 руб.
-
Мало5 490 руб. /шт7 490 руб.-27%Экономия 2 000 руб.
-
Много2 690 руб. /шт4 690 руб.-43%Экономия 2 000 руб.
-
Много2 190 руб. /шт3 190 руб.-31%Экономия 1 000 руб.
-
Достаточно2 390 руб. /шт3 390 руб.-29%Экономия 1 000 руб.
-
Много2 790 руб. /шт3 790 руб.-26%Экономия 1 000 руб.
-
Много3 490 руб. /шт5 490 руб.-36%Экономия 2 000 руб.
-
Мало2 690 руб. /шт3 690 руб.-27%Экономия 1 000 руб.
-
-
Много2 690 руб. /шт4 690 руб.-43%Экономия 2 000 руб.
-
Достаточно2 390 руб. /шт3 390 руб.-29%Экономия 1 000 руб.
-
Нет в наличии1 490 руб. /шт2 490 руб.-40%Экономия 1 000 руб.
-
Много2 490 руб. /шт3 490 руб.-29%Экономия 1 000 руб.
-
-
-
Мало2 690 руб. /шт4 690 руб.-43%Экономия 2 000 руб.
-
Много2 690 руб. /шт4 690 руб.-43%Экономия 2 000 руб.
-
Много2 790 руб. /шт3 790 руб.-26%Экономия 1 000 руб.
-
Нет в наличии1 490 руб. /шт2 490 руб.-40%Экономия 1 000 руб.
-
Много3 490 руб. /шт5 490 руб.-36%Экономия 2 000 руб.
-
Мало2 690 руб. /шт3 690 руб.-27%Экономия 1 000 руб.
Новости
Все новости
17 апреля 2023
Супер новинка электрошокер пистолет GUN PRO S уже в продаже
Уважаемые покупатели нашего интернет-магазина электрошокеров и других средств самообороны.
Новинка "Электрошокер пистолет GUN PRO S". ...
1 февраля 2021
Электрошокер Кастет по супер низкой цене
До наступления Нового года на шокер кастет действует специальная, праздничная цена — всего 1590 рублей! Успейте купить электрошокер в виде...
Подписывайтесь!
Узнавайте свежую информацию о скидках и акциях первым.
Действующие акции
Все акции
-
1 января 2023Акция скидка за репост в ВКУникальная возможность получить дополнительную скидку 10% на любой товар. Скидка за репост, подробности в описании
-
1 января 2021Электрошокер Кастет по супер низкой цене!До наступления Нового года на шокер кастет действует специальная, праздничная цена — всего 1790 рублей!
-
1 апреля 2020Три электрошокера по цене двухТолько этой весной при единовременной покупке 2-х электрошоков ОСА 1002 Vip Metallic (Super Light), вы получаете в подарок электрошокер ОСА ...
-
1 января 2020Акция два зарядных устройства по цене одного
Наш магазин объявляет для всех своих клиентов о начале новой акции «два товара по цене одного». Мы ценим наших покупателей и уверены в том,...
-
20 сентября 2018Купите фонарь электрошокер не только себе
До конца осени вы можете купить фонарь электрошокер Police ОСА 1101 в количестве двух штук, в подарок вы получите дополнительный электрошокер...
Наши магазины
Все магазины
-
Магазин в Санкт-ПетербургеАдрес: 192239 г. Санкт-Петербург, пер. Альпийский д. 18Телефон 8 (812) 448-64-37
-
Магазин в Ростове-на-ДонуАдрес: 344000 г. Ростов-на-Дону, пр. Ворошиловский д. 36-38Телефон 8-800-555-85-62
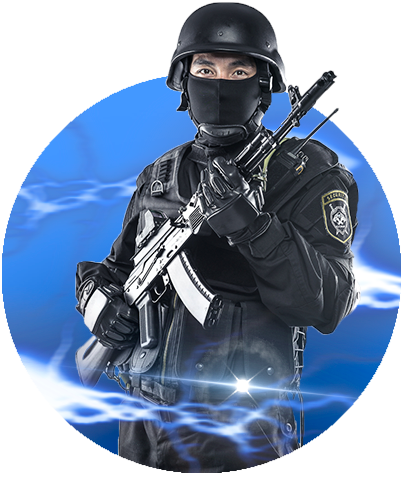
О компании
С 2011 года мы на рынке России. За прошедшие 14 лет мы существенно расширили ассортимент продукции и добавили новые разделы в нашем каталоге. Причины нашего успеха это: доверие клиентов, качественный товар, хороший сервис и конечно же – строго соблюдаемые взятые на себя гарантийные обязательства.
Наш каталог
Основная специализация нашего магазина – электрошокеры для самозащиты. Мы предлагаем все категории подобных устройств, а именно:
- контактные;
- дистанционные;
- замаскированные;
- комбинированные.
Подробнее